You can copy, or, duplicate whole indices, including records, settings, synonyms, and Rules.
You can copy indices within an Algolia application from the dashboard and the API.
If you want to copy an index between two Algolia applications, you can use the API.
For more information, see Copy indices between applications on this page.
Copying an index doesn’t copy the associated analytics data.
Copying an index is an expensive operations and is
rate-limited.
Copy indices from the Algolia dashboard
Copying an index duplicates the records and configuration,
including synonyms and Rules of an existing index (except the enableReRanking
setting).
To copy or duplicate an index in the dashboard:
- Go to the Algolia dashboard and select your index from the Index menu.
-
Select Manage index > Duplicate.
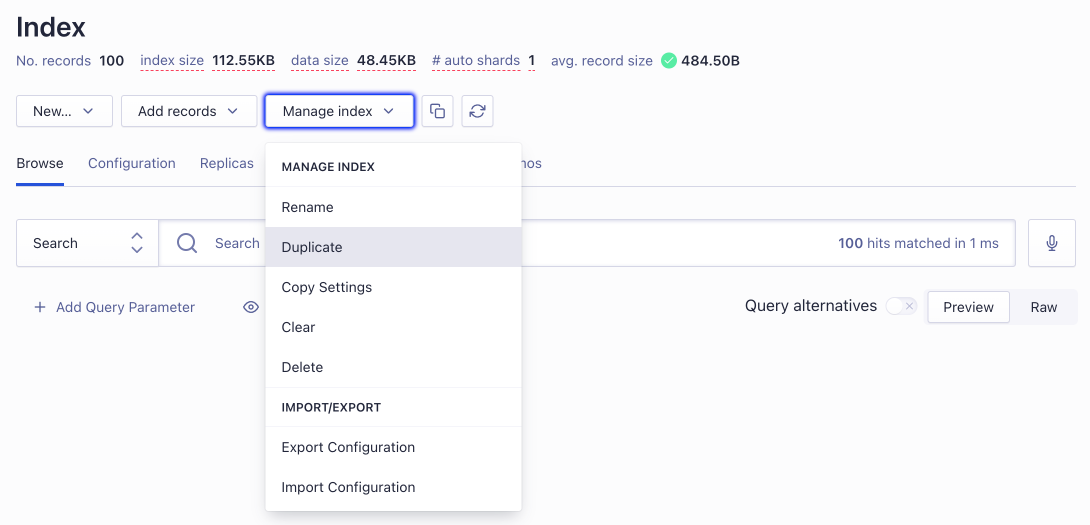
-
Select Create a new index and enter a new index name, if you want to copy an index to a new index.
Select Overwrite an existing index and enter an existing index name to overwrite an existing index.
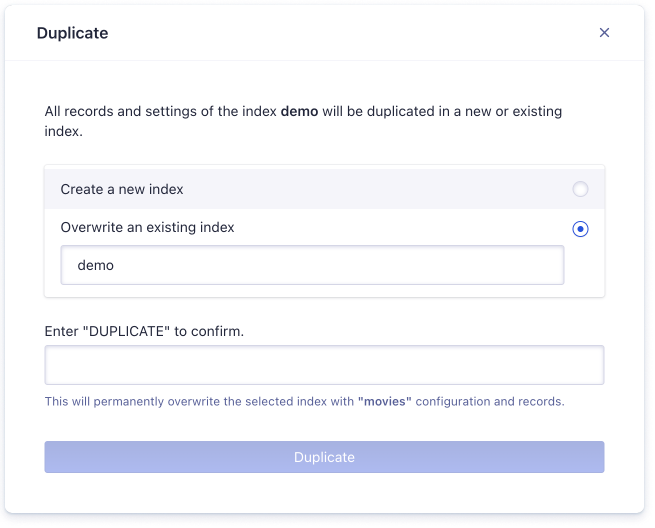
- Enter
DUPLICATE
to confirm (if you’re overwriting an existing index) and click Duplicate.
Copy indices using the API
To copy indices, use the copyIndex
method with an API client,
or the algolia indices copy
command with the Algolia CLI.
By providing a scope
parameter,
you can choose what you want to copy: records, settings, synonyms, or Rules.
Copying an index has no impact on Analytics, because you can’t copy an index’s analytics data.
The API keys of the source are merged with the existing keys in the destination index.
Copy indices fully
To copy an index including records, settings, synonyms, and Rules,
use the copyIndex
method without arguments:
1
2
3
4
5
6
7
8
9
10
11
| <?php
require_once __DIR__."/vendor/autoload.php";
use Algolia\AlgoliaSearch\SearchClient;
// Use an API key with `addObject` ACL
$client = SearchClient::create(
'YourApplicationID', 'YourAPIKey'
);
// Copy `indexNameSrc` to `indexNameDest`
$client->copyIndex('indexNameSrc', 'indexNameDest');
|
1
2
3
4
5
6
7
8
9
| require 'algolia'
# Use an API key with `addObject` ACL
client = Algolia::Search::Client.create(
'YourApplicationID', 'YourAPIKey'
)
# Copy `indexNameSrc` to `indexNameDest`
client.copy_index('indexNameSrc', 'indexNameDest')
|
1
2
3
4
5
6
7
| const algoliasearch = require('algoliasearch');
// Use an API key with `addObject` ACL
const client = algoliasearch('YourApplicationID', 'YourAPIKey');
// Copy `indexNameSrc` to `indexNameDest`
client.copyIndex('indexNameSrc', 'indexNameDest');
|
1
2
3
4
5
6
7
| from algoliasearch.search_client import SearchClient
# Use an API key with `addObject` ACL
client = SearchClient.create("YourApplicationID", "YourAPIKey")
# Copy `indexNameSrc` to `indexNameDest`
client.copy_index("indexNameSrc", "indexNameDest")
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| import AlgoliaSearchClient
// Use an API key with `addObject` ACL
let client = SearchClient(
appID: "YourApplicationID",
apiKey: "YourAPIKey"
)
// Copy `indexNameSrc` to `indexNameDest`
client.copyIndex(from: "indexNameSrc", to: "indexNameDest") { result in
if case .success(let response) = result {
print("Response: \(response)")
}
}
|
1
2
3
4
5
6
7
8
| // Use an API key with `addObject` ACL
val client = ClientSearch(
ApplicationID("YourApplicationID")
APIKey("YourAPIKey")
)
// Copy `indexNameSrc` to `indexNameDest`
val index = client.initIndex(IndexName("indexNameSrc"))
index.copyIndex(IndexName("indexNameDest"))
|
1
2
3
4
5
6
7
8
| // Use an API key with `addObject` ACL
var client = new SearchClient("YourApplicationID", "YourAPIKey");
// Copy `indexNameSrc` to `indexNameDest`
client.CopyIndex("indexNameSrc", "indexNameDest");
// Asynchronous
await client.CopyIndexAsync("indexNameSrc", "indexNameDest");
|
1
2
3
4
5
6
7
8
| // Use an API key with `addObject` ACL
SearchClient client = DefaultSearchClient.create("YourApplicationID", "YourAPIKey");
// Copy `indexNameSrc` to `indexNameDest`
client.copyIndex("indexNameSrc", "indexNameDest");
// Asynchronous
client.copyIndexAsync("indexNameSrc", "indexNameDest");
|
1
2
3
4
5
| // Use an API key with `addObject` ACL
client := search.NewClient("YourApplicationID", "YourAPIKey")
// Copy `indexNameSrc` to `indexNameDest`
res, err := client.CopyIndex("indexNameSrc", "indexNameDest")
|
1
2
3
4
5
| // Use an API key with `addObject` ACL
val client = new AlgoliaClient("YourApplicationID", "YourAPIKey")
// Copy `indexNameSrc` to `indexNameDest`
client.execute { copy index "indexNameSrc" to "indexNameDest" }
|
1
2
| # Copy `indexNameSrc` to `indexNameDest`
algolia index copy <indexNameSrc> <indexNameDest>
|
If an index with the new name already exists, it’s overwritten after the renaming.
To prevent accidentally overwriting existing indices,
you can check if an index exists with the indexExists
method.
If the source index doesn’t exist, copyIndex
creates an empty index with zero records under the new name.
To prevent this, you can also use the indexExists
method to see if the source index exists before copying.
Copy indices partially
To copy parts of an index, you can use the scope
parameter.
For example, to copy only the synonyms and settings (but not records or Rules) between indices:
1
2
3
4
5
6
7
8
9
10
11
12
13
| <?php
require_once __DIR__."/vendor/autoload.php";
use Algolia\AlgoliaSearch\SearchClient;
// Use an API key with `addObject` ACL
$client = SearchClient::create(
'YourApplicationID', 'YourAPIKey'
);
// Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
$client->copyIndex('indexNameSrc', 'indexNameDest', [
'scope' => ['settings', 'synonyms']
]);
|
1
2
3
4
5
6
7
8
9
10
11
| require 'algolia'
# Use an API key with `addObject` ACL
client = Algolia::Search::Client.create(
'YourApplicationID', 'YourAPIKey'
)
# Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
client.copy_index('indexNameSrc', 'indexNameDest', {
scope: ['settings', 'synonyms']
})
|
1
2
3
4
| // Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
client.copyIndex('indexNameSrc', 'indexNameDest', {
scope: ['settings', 'synonyms']
});
|
1
2
3
4
5
6
7
8
9
| from algoliasearch.search_client import SearchClient
# Use an API key with `addObject` ACL
client = SearchClient.create("YourApplicationID", "YourAPIKey")
# Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
client.copy_index("indexNameSrc", "indexNameDest", {
"scope": ["settings", "synonyms"]
})
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| import AlgoliaSearchClient
// Use an API key with `addObject` ACL
let client = SearchClient(
appID: "YourApplicationID",
apiKey: "YourAPIKey"
)
// Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
client.copyIndex(
from: "indexNameSrc",
to: "indexNameDest",
scope: [Scope.settings, Scope.synonyms]
)
|
1
2
3
4
5
6
7
8
9
10
11
12
13
| // Use an API key with `addObject` ACL
val client = ClientSearch(
ApplicationID("YourApplicationID"),
APIKey("YourAPIKey")
)
// Copy only synonyms and settings from `indexNameSrc` to `indexNameDest`
val index = client.initIndex(IndexName("indexNameSrc"))
val scopes = listOf(
Scope.Settings,
Scope.Synonyms
)
index.copyIndex(IndexName("indexNameDest"), scopes)
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| // Use an API key with `addObject` ACL
var client = new SearchClient("YourApplicationID", "YourAPIKey");
var index = client.InitIndex("indexNameSrc");
// Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
List<CopyScope> scopes = new List<CopyScope>() {
CopyScope.SETTINGS,
CopyScope.SYNONYMS
};
index.CopyTo("indexNameDest", scope: scopes);
// Asynchronous
originalIndex.CopyToAsync("indexNameDest", scope: scopes);
|
1
2
3
4
5
6
7
8
| // Use an API key with `addObject` ACL
SearchClient client = DefaultSearchClient.create("YourApplicationID", "YourAPIKey");
// Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
client.copyIndex(
"indexNameSrc", "indexNameDest",
Arrays.asList("settings", "synonyms")
);
|
1
2
3
4
5
6
7
8
9
| // Use an API key with `addObject` ACL
client := search.NewClient("YourApplicationID", "YourAPIKey")
// Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
res, err := client.CopyIndex(
"indexNameSrc",
"indexNameDest",
opt.Scopes("settings", "synonyms"),
)
|
1
2
3
4
5
6
7
| // Use an API key with `addObject` ACL
val client = new AlgoliaClient("YourApplicationID", "YourAPIKey")
// Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
client.execute {
copy index "indexNameSrc" to "indexNameDest" scopes Seq("settings", "synonyms")
}
|
1
2
3
| # Copy only settings and synonyms from `indexNameSrc` to `indexNameDest`
algolia index copy <indexNameSrc> <indexNameDest> \
--scopes "settings,synonyms"
|
If you use the scope
parameter, records aren’t copied to the new index.
Existing items of the scope are replaced. Items belonging to other scopes are preserved.
For example, if you use the settings
scope, only the settings are copied to the destination index,
keeping the records, synonyms, and Rules.
See Scopes for more information.
Copying indices with replicas
Copying a source index that has replicas copies the index’s data, but not its replicas.
The destination index won’t have replicas.
You can’t copy to a destination index that already has replicas.
Copy indices between different applications
To copy an index between different Algolia applications,
use the AccountClient
, which is part of the Algolia API clients,
or you can use the Algolia CLI.
For JavaScript, the AccountClient
is distributed as a separate package @algolia/client-account
.
You can install it from npm:
1
| npm install @algolia/client-account
|
1
2
3
4
5
6
7
8
| use \Algolia\AlgoliaSearch\SearchClient;
use \Algolia\AlgoliaSearch\AccountClient;
// Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
$sourceIndex = SearchClient::create('SOURCE_APP_ID', 'SOURCE_API_KEY')->initIndex('indexNameSrc');
$targetIndex = SearchClient::create('TARGET_APP_ID', 'TARGET_API_KEY')->initIndex('indexNameDest');
AccountClient::copyIndex($sourceIndex, $targetIndex);
|
1
2
3
4
5
6
7
8
9
10
11
| require 'algolia'
# Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
source_index = Algolia::Search::Client.create(
'SOURCE_APP_ID', 'SOURCE_API_KEY'
).init_index('indexNameSrc')
target_index = Algolia::Search::Client.create(
'TARGET_APP_ID', 'TARGET_API_KEY'
).init_index('indexNameDest')
Algolia::Account::Client.copy_index(source_index, target_index)
|
1
2
3
4
5
6
7
8
| const algoliasearch = require('algoliasearch');
const accountCopyIndex = require('@algolia/client-account').accountCopyIndex;
// Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
const sourceIndex = algoliasearch('SOURCE_APP_ID', 'SOURCE_API_KEY').initIndex('indexNameSrc');
const targetIndex = algoliasearch('TARGET_APP_ID', 'TARGET_API_KEY').initIndex('indexNameDest');
accountCopyIndex(sourceIndex, targetIndex);
|
1
2
3
4
5
6
7
8
9
10
11
12
13
| from algoliasearch.search_client import SearchClient
from algoliasearch.account_client import AccountClient
# Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
source_index = SearchClient.create(
"SOURCE_APP_ID", "SOURCE_API_KEY"
).init_index("indexNameSrc")
target_index = SearchClient.create(
"TARGET_APP_ID", "TARGET_API_KEY"
).init_index("indexNameDest")
AccountClient.copy_index(source_index, target_index)
|
1
2
3
4
5
6
7
8
9
10
11
| import AlgoliaSearchClient
// Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
let sourceIndex = SearchClient(appID: "SOURCE_APP_ID", apiKey: "SOURCE_API_KEY").index(withName: "indexNameSrc")
let targetIndex = SearchClient(appID: "TARGET_APP_ID", apiKey: "TARGET_API_KEY").index(withName: "indexNameDest")
try AccountClient.copyIndex(source: sourceIndex, destination: targetIndex) { result in
if case .success(let response) = result {
print("Response: \(response)")
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
| // Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
val sourceIndex = ClientSearch(
ApplicationID("SOURCE_APP_ID"),
APIKey("SOURCE_API_KEY")
).initIndex(IndexName("indexNameSrc"))
val targetIndex = ClientSearch(
ApplicationID("TARGET_APP_ID"),
APIKey("TARGET_API_KEY")
).initIndex(IndexName("indexNameDest"))
ClientAccount.copyIndex(sourceIndex, targetIndex)
|
1
2
3
4
5
6
7
8
9
10
11
| // Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
SearchClient sourceClient = new SearchClient("SOURCE_APP_ID", "SOURCE_API_KEY");
SearchClient targetClient = new SearchClient("TARGET_APP_ID", "TARGET_API_KEY");
SearchIndex sourceIndex = sourceClient.initIndex("indexNameSrc");
SearchIndex targetIndex = targetClient.initIndex("indexNameDest");
AccountClient.copyIndex(sourceIndex, targetIndex);
// Asynchronous
AccountClient.copyIndexAsync(sourceIndex, destinationIndex);
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
| // Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
SearchClient sourceClient =
DefaultSearchClient.create("SOURCE_APP_ID", "SOURCE_API_KEY");
SearchIndex<Record> sourceIndex =
sourceClient.initIndex("indexNameSrc", Record.class);
SearchClient targetClient =
DefaultSearchClient.create("TARGET_APP_ID", "TARGET_API_KEY");
SearchIndex<Record> targetIndex =
targetClient.initIndex("indexNameDest", Record.class);
AccountClient.copyIndex(sourceIndex, targetIndex);
// Asynchronous
AccountClient.copyIndexAsync(sourceIndex, destinationIndex);
|
1
2
3
4
5
| // Copy `indexNameSrc` from app `SOURCE_APP_ID` to app `TARGET_APP_ID`
sourceIndex = search.NewClient("SOURCE_APP_ID", "SOURCE_API_KEY").InitIndex("indexNameSrc")
targetIndex = search.NewClient("TARGET_APP_ID", "TARGET_API_KEY").InitIndex("indexNameDest")
res, err := search.NewAccount().CopyIndex(sourceIndex, targetIndex)
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
| # Copy records from `indexNameSrc` of the profile `SOURCE` to `indexNameDest` of the profile `TARGET`
algolia --profile <SOURCE> objects browse <indexNameSrc> \
| algolia --profile <TARGET> objects import <indexNameDest> --file -
# Copy settings
algolia --profile <SOURCE> settings get <indexNameSrc> \
| algolia --profile <TARGET> settings import <indexNameDest> --settings-file -
# Copy synonyms
algolia --profile <SOURCE> synonyms browse <indexNameSrc> \
| algolia --profile <TARGET> synonyms import <indexNameDest> --file -
# Copy Rules
algolia --profile <SOURCE> rules browse <indexNameSrc> \
| algolia --profile <TARGET> rules import <indexNameDest> --file -
|
If you want to move an index between Algolia applications,
you can copy it first, and then delete it.
Copy index settings
If you want to copy only an index’s setting to another index, you can use the
Manage index > Copy Settings action in the dashboard,
or the copySettings
method of the API clients.