Deleting an index permanently removes the records and the index configuration,
like searchable attributes and custom ranking, from your Algolia application.
Instead of deleting the complete index, you can also delete (clear) just the records,
keeping the configuration.
See Clear records from an index on this page for more information.
Before you delete an index, you may want to create a backup by exporting your index.
Deleting an index doesn’t affect its analytics data.
Deleting indices with replicas
If the index you want to delete is a replica of another index,
you must first unlink it.
If the index is a primary index and has replicas,
the replica indices will become independent indices if you delete their primary index.
Delete indices from the Algolia dashboard
To delete an index from the dashboard:
- Go to the Algolia dashboard and select your index from the Index menu.
-
Select Manage index > Delete.
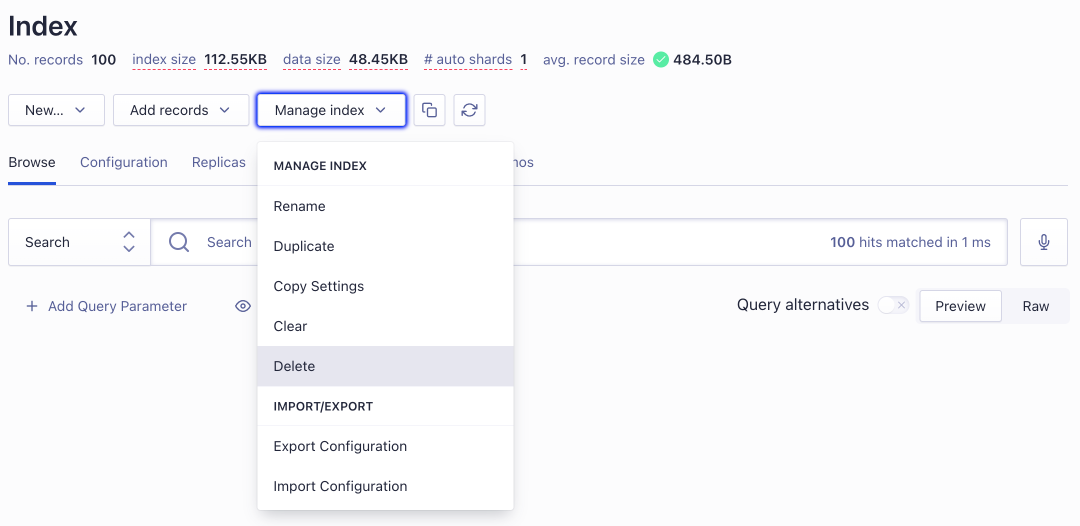
- Type
DELETE
to confirm and click Delete.
Delete indices with the API
To delete an index, use the deleteIndex
method with an API client
or the algolia indices delete
command with the Algolia CLI.
1
2
3
4
5
6
7
8
9
10
| <?php
require_once __DIR__."/vendor/autoload.php";
use Algolia\AlgoliaSearch\SearchClient;
// Use an API key with `deleteIndex` ACL
$client = SearchClient::create(
'YourApplicationID', 'YourAPIKey'
);
$index = $client->initIndex('indexName');
$index->delete();
|
1
2
3
4
5
6
7
8
| require 'algolia'
# Use an API key with `deleteIndex` ACL
client = Algolia::Search::Client.create(
'YourApplicationID', 'YourAPIKey'
)
index = client.init_index('indexName')
index.delete
|
1
2
3
4
5
6
7
| const algoliasearch = require('algoliasearch');
// Use an API key with `deleteIndex` ACL
const client = algoliasearch('YourApplicationID', 'YourAPIKey');
const index = client.initIndex('indexName');
index.delete();
|
1
2
3
4
5
6
| from algoliasearch.search_client import SearchClient
# Use an API key with `deleteIndex` ACL
client = SearchClient.create("YourApplicationID", "YourAPIKey")
index = client.init_index("indexName")
index.delete()
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| import AlgoliaSearchClient
// Use an API key with `deleteIndex` ACL
let client = SearchClient(
appID: "YourApplicationID",
apiKey: "YourAPIKey"
)
let index = client.index(withName: "indexName")
index.delete { result in
if case .success(let response) = result {
print("Response: \(response)")
}
}
|
1
2
3
4
5
6
7
8
| // Use an API key with `deleteIndex` ACL
val client = ClientSearch(
ApplicationID("YourApplicationID"),
APIKey("YourAPIKey")
)
val index = client.initIndex(IndexName("indexName"))
index.deleteIndex()
|
1
2
3
4
5
6
7
8
| // Use an API key with `deleteIndex` ACL
var client = new SearchClient("YourApplicationID", "YourAPIKey");
var index = client.InitIndex("indexName");
index.Delete();
// Asynchronous
await index.DeleteAsync();
|
1
2
3
4
5
6
7
8
| // Use an API key with `deleteIndex` ACL
SearchClient client = DefaultSearchClient.create("YourApplicationID", "YourAPIKey");
SearchIndex<Record> index = client.initIndex("indexName", Record.class);
index.delete();
//Asynchronous
index.deleteAsync();
|
1
2
3
4
| // Use an API key with `deleteIndex` ACL
client := search.NewClient('YourApplicationID', 'YourAPIKey')
index := client.InitIndex('indexName')
res, err := index.Delete()
|
1
2
3
| // Use an API key with `deleteIndex` ACL
val client = new AlgoliaClient("YourApplicationID", "YourAPIKey")
client.execute { delete index "index" }
|
1
| algolia index delete <indexName>
|
Using API clients, you can only delete one index at a time.
Delete multiple indices
To delete more than one index, use listIndices
to get an application’s indices and then use the multipleBatch
method to delete multiple indices with a single API call.
If you want to delete replica indices, you have to delete their primary indices first.
You can delete multiple indices with the algolia indices delete
command.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
| <?php
require_once __DIR__."/vendor/autoload.php";
use Algolia\AlgoliaSearch\SearchClient;
// You need an API key with `deleteIndex` permissions
$client = SearchClient::create('YourApplicationID', 'YourAPIKey');
// List all indices
$indices = $client->listIndices();
// Primary indices don't have a `primary` key
$primaryIndices = array_filter($indices['items'], function ($index){
return !isset($index['primary']);
});
$replicaIndices = array_filter($indices['items'], function ($index){
return !isset($index['primary']);
});
// Delete primary indices first
foreach ($primaryIndices as $i) {
$index = $client->initIndex($i['name']);
$index->delete()->wait();
}
echo "Deleted primary indices.\n";
// Now, delete replica indices
foreach ($replicaIndices as $i) {
$index = $client.initIndex($i['name']);
$index->delete()->wait();
}
echo "Deleted replica indices.\n";
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
| require 'algolia'
# You need an API key with `deleteIndex` permissions
client = Algolia::Search::Client.create('YourAppplicationId', 'YourAPIKey')
# List all indices
indices = client.list_indexes
# Primary indices don't have a `primary` key
primaryIndices, replicaIndices = indices[:items].partition { |index| index[:primary].nil? }
# Delete primary indices first
client.multiple_batch(
primaryIndices.map { |index| { indexName: index[:name], action: 'delete' } }
).wait
puts 'Deleted primary indices.'
# Now, delete replica indices
client.multiple_batch(
replicaIndices.map { |index| { indexName: index[:name], action: 'delete' } }
)
puts 'Deleted replica indices.'
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
| const algoliasearch = require("algoliasearch");
// You need an API key with `deleteIndex` permissions
const client = algoliasearch("YourApplicationID", "YourAPIKey");
// Use async/await syntax
(async () => {
// List all indices
const indices = await client.listIndices();
// Primary indices don't have a `primary` key
const primaryIndices = indices.items.filter((index) => !index.primary);
const replicaIndices = indices.items.filter((index) => index.primary);
// Delete primary indices first
client
.multipleBatch(
primaryIndices.map((index) => {
return { indexName: index.name, action: "delete" };
})
)
.wait();
console.log("Deleted primary indices.");
// Now, delete replica indices
client.multipleBatch(
replicaIndices.map((index) => {
return { indexName: index.name, action: "delete" };
})
);
console.log("Deleted replica indices.");
})();
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
| from algoliasearch.search_client import SearchClient
# You need an API key with `deleteIndex` permissions
client = SearchClient.create('YourApplicationID', 'YourAPIKey')
# List all indices
indices = client.list_indices()
primaries = []
replicas = []
for index in indices["items"]:
action = {"indexName": index["name"], "action": "delete"}
# Primary indices don't have a `primary` key
if not "primary" in index :
primaries.append(action)
else:
replicas.append(action)
# Delete primary indices first
client.multiple_batch(primaries).wait()
print("Deleted primary indices.")
# Now, delete replica indices
client.multiple_batch(replicas)
print("Deleted replica indices.")
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
| import AlgoliaSearchClient
// You need an API key with `deleteIndex`
let client = SearchClient(
appID: "YourApplicationID",
apiKey: "YourAPIKey"
)
// List all indices
let indices = try client.listIndices()
// Primary indices don't have a `primary` key
let primaryIndices = indices.items.filter { $0.primary == nil }
let replicaIndices = indices.items.filter { $0.primary != nil }
// Delete primary indices first
try! client.multipleBatchObjects(operations: primaryIndices)
print("Deleted primary indices.")
// Now, delete replica indices
try! client.multipleBatchObjects(operations: replicaIndices)
print("Deleted replica indices.")
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
| import com.algolia.search.client.ClientSearch
import com.algolia.search.model.APIKey
import com.algolia.search.model.ApplicationID
import com.algolia.search.model.indexing.BatchOperation
import com.algolia.search.model.multipleindex.BatchOperationIndex
suspend fun main() {
// You need an API key with `deleteIndex` permission
val client = ClientSearch(
applicationID = ApplicationID("YourApplicationID"),
apiKey = APIKey("YourAPIKey")
)
// Primary indices don't have a `primary` key
val (primaryIndices, replicaIndices) = client.listIndices().items.partition {
it.primaryOrNull == null
}
// Delete primary indices first
if (primaryIndices.isNotEmpty()) {
client.multipleBatchObjects(
primaryIndices.map {
BatchOperationIndex(it.indexName, BatchOperation.DeleteIndex)
}
)
println("Deleted primary indices.")
}
// Now, delete replica indices
if (replicaIndices.isNotEmpty()) {
client.multipleBatchObjects(
replicaIndices.map {
BatchOperationIndex(it.indexName, BatchOperation.DeleteIndex)
}
)
println("Deleted replica indices.")
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
| using System;
using System.Collections.Generic;
using Algolia.Search.Clients;
using Algolia.Search.Models.Batch;
using Algolia.Search.Models.Enums;
namespace DeleteIndex
{
class Program
{
static void Main(string[] args)
{
// You need an API key with `deleteIndex` permissions
var client = new SearchClient(
"YourApplicationID",
"YourAPIKey"
);
// List all indices
var indices = client.ListIndices().Items;
var primaryIndices = new List<BatchOperation<string>>();
var replicaIndices = new List<BatchOperation<string>>();
foreach (var index in indices)
{
var action = new BatchOperation<string>
{
IndexName = index.Name,
Action = BatchActionType.Delete
};
// Primary indices don't have a `Primary` key
if (index.Primary == null)
{
primaryIndices.Add(action);
}
else
{
replicaIndices.Add(action);
}
}
// Delete primary indices first
if (primaryIndices.Count > 0)
{
client.MultipleBatch(primaryIndices).Wait();
Console.WriteLine("Deleted primary indices.");
}
// Now, delete replica indices
if (replicaIndices.Count > 0)
{
client.MultipleBatch(replicaIndices);
Console.WriteLine("Deleted replica indices.");
}
}
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
| import com.algolia.search.DefaultSearchClient;
import com.algolia.search.SearchClient;
import com.algolia.search.models.indexing.ActionEnum;
import com.algolia.search.models.indexing.BatchOperation;
import com.algolia.search.models.indexing.IndicesResponse;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class Program {
public static void main(String[] args) {
// You need an API key with `deleteIndex` permissions
SearchClient client = DefaultSearchClient.create(
"YourApplicationID", "YourAPIKey"
);
// List indices, primary indices don't have a `primary` key
Map<Boolean, List<IndicesResponse>> indices = client.listIndices().stream().collect(Collectors.partitioningBy(index -> index.getPrimary() == null));
List<IndicesResponse> primaryIndices = indices.get(true);
List<IndicesResponse> replicaIndices = indices.get(false);
// Delete primary indices first
if (!primaryIndices.isEmpty()) {
client.multipleBatch(
primaryIndices
.stream()
.map(index -> new BatchOperation<>(index.getName(), ActionEnum.DELETE))
.collect(Collectors.toList()));
System.out.println("Deleted primary indices.");
}
// Now, delete replica indices
if (!replicaIndices.isEmpty()) {
client.multipleBatch(
replicaIndices
.stream()
.map(index -> new BatchOperation<>(index.getName(), ActionEnum.DELETE))
.collect(Collectors.toList()));
System.out.println("Deleted replica indices.");
}
System.exit(0);
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
| package main
import (
"fmt"
"github.com/algolia/algoliasearch-client-go/v3/algolia/search"
)
func main() {
// You need an API key with `deleteIndex` permissions
client := search.NewClient(
"YourApplicationID",
"YourAPIKey"
)
// List all indices
indices, _ := client.ListIndices()
var primaryIndices []search.BatchOperationIndexed
var replicaIndices []search.BatchOperationIndexed
for _, index := range indices.Items {
action := search.BatchOperationIndexed{
IndexName: index.Name,
BatchOperation: search.BatchOperation{Action: search.Delete},
}
// Primary indices don't have a `Primary` key
if index.Primary == "" {
primaryIndices = append(primaryIndices, action)
} else {
replicaIndices = append(replicaIndices, action)
}
}
if len(primaryIndices) > 0 {
res, err := client.MultipleBatch(primaryIndices)
if err != nil {
panic(err)
}
res.Wait()
fmt.Println("Primary indices deleted")
}
if len(replicaIndices) > 0 {
_, err := client.MultipleBatch(replicaIndices)
if err != nil {
panic(err)
}
fmt.Println("Replica indices deleted")
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
| package org.example
import algolia.AlgoliaClient
import algolia.AlgoliaDsl._
import algolia.responses.Indices
import scala.concurrent.Await
import scala.concurrent.ExecutionContext.Implicits.global
import scala.concurrent.duration.Duration
import scala.language.postfixOps
object Program extends App {
// You need an API key with `deleteIndex` permission
val client = new AlgoliaClient("YourApplicationID", "YourAPIKey")
// List all indices
val indices: Indices = Await.result(
client.execute(list indices), Duration.Inf
)
// Primary indices don't have a `primary` key
val (primaryIndices, replicaIndices) = indices.items.partition(_.primary == null)
// Delete primary indices first
for (i <- primaryIndices.items) {
Await.result(client.execute(delete index i.name), Duration.Inf)
}
println("Deleted primary indices.")
// Now, delete replica indices
for (i <- replicaIndices.items) {
Await.result(client.execute(delete index i.name), Duration.Inf)
}
println("Deleted replica indices.")
}
|
1
| $ algolia index delete <INDEX_1> <INDEX_2> <INDEX_3>
|
Clear records from an index using the Algolia dashboard
If you only want to delete (clear) the records from an index, keeping the index configuration:
-
Go to the Algolia dashboard and select your index from the Index menu.
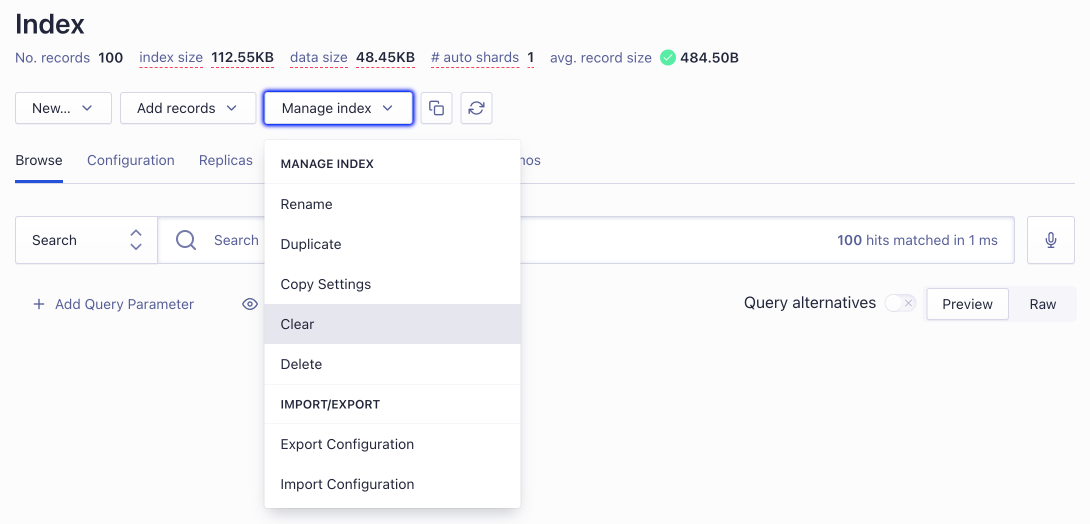
-
Type CLEAR
to confirm and click Clear.
Clear records from an index using the API
To remove only the records from the index,
while keeping the settings, synonyms, and Rules,
use the clearObjects
method with an API client
or the algolia indices clear
command .
1
2
3
4
5
6
7
8
9
10
| <?php
require_once __DIR__."/vendor/autoload.php";
use Algolia\AlgoliaSearch\SearchClient;
// You need an API key with `deleteIndex` permissions
$client = SearchClient::create('YourApplicationID', 'YourAPIKey');
$index = $client->initIndex('YourIndexName');
$index->clearObjects();
echo "Deleted records.\n";
|
1
2
3
4
5
6
7
8
| require 'algolia'
# You need an API key with `deleteIndex` permissions
client = Algolia::Search::Client.create('YourAppplicationID', 'YourAPIKey')
index = client.init_index('YourIndexName')
index.clear_objects
puts "Deleted records."
|
1
2
3
4
5
6
7
8
| const algoliasearch = require("algoliasearch");
// You need an API key with `deleteIndex` permissions
const client = algoliasearch("YourApplicationID", "YourAPIKey");
const index = client.initIndex("YourIndexName");
index.clearObjects();
console.log("Deleted records");
|
1
2
3
4
5
6
7
8
| from algoliasearch.search_client import SearchClient
# You need an API key with `deleteIndex` permissions
client = SearchClient.create("YourApplicationID", "YourAPIKey")
index = client.init_index("YourIndexName")
index.clear_objects()
print("Deleted records.")
|
1
2
3
4
5
6
7
8
9
10
| import AlgoliaSearchClient
let client = SearchClient(
appID: "YourApplicationID",
apiKey: "YourAPIKey"
)
let index = client.index(withName: "YourIndexName")
try! index.clearObjects()
print("Deleted records.")
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| import com.algolia.search.client.ClientSearch
import com.algolia.search.model.APIKey
import com.algolia.search.model.ApplicationID
import com.algolia.search.model.IndexName
suspend fun main() {
// You need an API key with `deleteIndices` permission
val client = ClientSearch(
applicationID = ApplicationID("YourApplicationID"),
apiKey = APIKey("YourAPIKey")
)
val index = client.initIndex(IndexName("YourIndexName"))
index.clearObjects()
println("Deleted records.")
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
| using System;
using Algolia.Search.Clients;
namespace ClearObjects
{
class Program
{
static void Main(string[] args)
{
// You need an API key with `deleteIndex` permissions
var client = new SearchClient(
"YourApplicationID",
"YourAPIKey"
);
var index = client.InitIndex("YourIndexName");
index.ClearObjects();
Console.WriteLine("Deleted records.");
}
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| import com.algolia.search.DefaultSearchClient;
import com.algolia.search.SearchClient;
import com.algolia.search.SearchIndex;
public class Program {
public static void main(String[] args) {
// You need an API key with `deleteIndex` permissions
SearchClient client = DefaultSearchClient.create(
"YourApplicationID", "YourAPIKey"
);
SearchIndex index = client.initIndex("YourIndexName");
index.clearObjects();
System.out.println("Deleted records.");
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
| package main
import (
"fmt"
"github.com/algolia/algoliasearch-client-go/v3/algolia/search"
)
func main() {
// You need an API key with `deleteIndex` permissions
client := search.NewClient(
"YourApplicationID",
"YourAPIKey"
)
index := client.InitIndex("YourIndexName")
index.ClearObjects()
fmt.Println("Deleted records.")
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
| package org.example
import algolia.AlgoliaClient
import algolia.AlgoliaDsl._
import scala.concurrent.Await
import scala.concurrent.ExecutionContext.Implicits.global
import scala.concurrent.duration.Duration
import scala.language.postfixOps
object Program extends App {
// You need an API key with `deleteIndex` permissions
val client = new AlgoliaClient("YourApplicationID", "YourAPIKey")
Await.result(
client.execute(clear index "YourIndexName"), Duration.Inf
)
println("Deleted records.")
}
|
1
| $ algolia index clear YourIndexName
|