If you want to rename indices, you can move them using the Algolia dashboard or the API.
Moving an index doesn’t move the associated analytics data.
You can only rename primary indices.
See Rename replica indices for more information.
Moving or renaming an index is an expensive operation and is
rate-limited.
Rename indices from the Algolia dashboard
To rename or move an index in the dashboard:
-
Go to the Algolia dashboard and select your index from the Index menu.
-
Select Manage index > Rename.
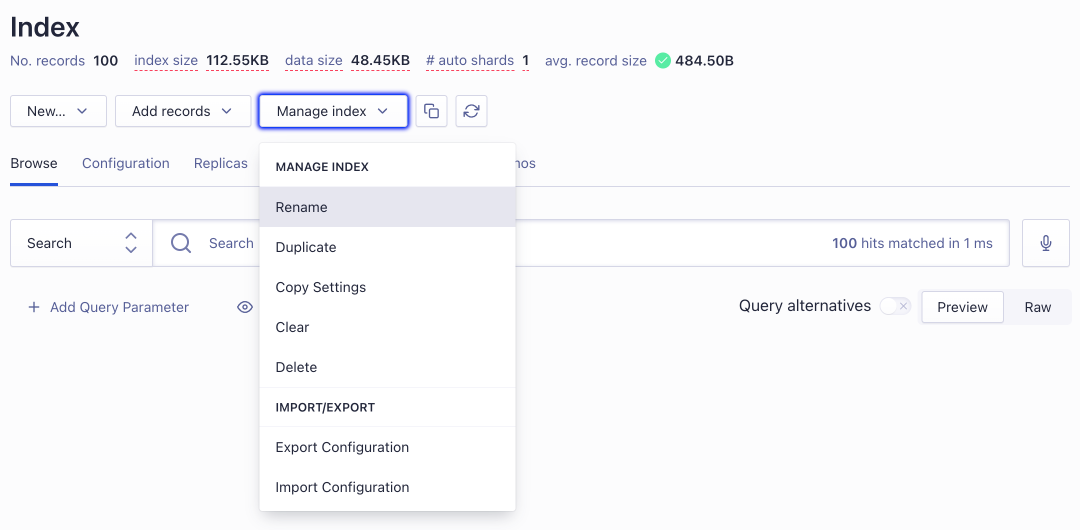
The Rename option only shows if you selected a primary index.
It doesn’t show for replica indices.
-
Enter the current and new index names and click Rename.
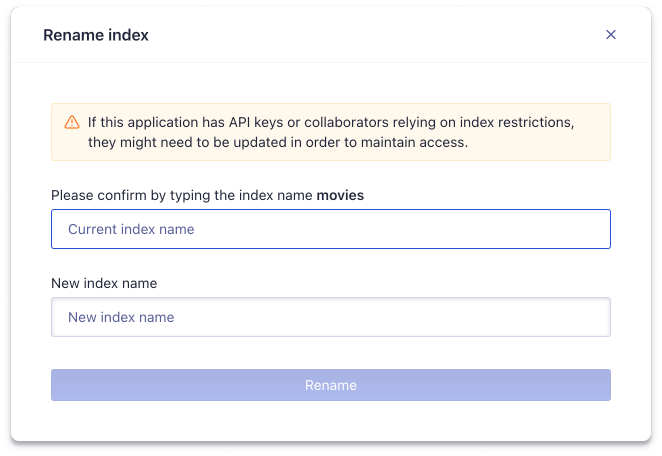
If an index with the new name already exists, it’s overwritten.
Rename indices using the API
To rename or move an index, use the moveIndex
method with an API client,
or use the algolia indices move
command with the Algolia CLI.
To move an index between different Algolia applications,
see Copy indices between
apps.
1
2
3
4
5
6
7
8
9
10
11
| <?php
require_once __DIR__."/vendor/autoload.php";
use Algolia\AlgoliaSearch\SearchClient;
// Use an API key with `addObject` ACL
$client = SearchClient::create(
'YourApplicationID', 'YourAPIKey'
);
// Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
$index = $client->moveIndex('indexNameSrc', 'indexNameDest');
|
1
2
3
4
5
6
7
8
9
| require 'algolia'
# Use an API key with `addObject` ACL
client = Algolia::Search::Client.create(
'YourApplicationID', 'YourAPIKey'
)
# Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
client.move_index('indexNameSrc', 'indexNameDest')
|
1
2
3
4
5
6
7
| const algoliasearch = require('algoliasearch');
// Use an API key with `addObject` ACL
const client = algoliasearch('YourApplicationID', 'YourAPIKey');
// Rename indexNameSrc to indexNameDest (and overwrite it)
client.moveIndex('indexNameSrc', 'indexNameDest');
|
1
2
3
4
5
6
7
| from algoliasearch.search_client import SearchClient
# Use an API key with `addObject` ACL
client = SearchClient.create("YourApplicationID", "YourAPIKey")
# Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
client.move_index("indexNameSrc", "indexNameDest")
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| import AlgoliaSearchClient
// Use an API key with `addObject` ACL
let client = SearchClient(
appID: "YourApplicationID",
apiKey: "YourAPIKey"
)
// Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
client.moveIndex(from: "indexNameSrc", to: "indexNameDest") { result in
if case .success(let response) = result {
print("Response: \(response)")
}
}
|
1
2
3
4
5
6
7
8
| // Use an API key with `addObject` ACL
val client = ClientSearch(
ApplicationID("YourApplicationID")
APIKey("YourAPIKey")
)
// Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
val index = client.initIndex(IndexName("indexNameSrc"))
index.moveIndex(IndexName("indexNameDest"))
|
1
2
3
4
5
6
7
8
| // Use an API key with `addObject` ACL
var client = new SearchClient("YourApplicationID", "YourAPIKey");
// Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
client.MoveIndex("indexNameSrc", "indexNameDest");
// Asynchronous
await client.MoveIndexAsync("indexNameSrc", "indexNameDest");
|
1
2
3
4
5
6
7
8
| // Use an API key with `addObject` ACL
SearchClient client = DefaultSearchClient.create("YourApplicationID", "YourAPIKey");
// Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
client.moveIndex("indexNameSrc", "indexNameDest");
// Asynchronous
client.moveIndexAsync("indexNameSrc", "indexNameDest");
|
1
2
3
4
5
| // Use an API key with `addObject` ACL
client := search.NewClient("YourApplicationID", "YourAPIKey")
// Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
res, err := client.MoveIndex("indexNameSrc", "indexNameDest")
|
1
2
3
4
5
| // Use an API key with `addObject` ACL
val client = new AlgoliaClient("YourApplicationID", "YourAPIKey")
// Rename `indexNameSrc` to `indexNameDest` (and overwrite it)
client.execute { move index "indexNameSrc" to "indexNameDest" }
|
1
2
| # Rename indexNameSrc to indexNameDest (and overwrite it)
algolia indices move <indexNameSrc> <indexNameDest>
|
If an index with the new name already exists, move
overwrites it.
To prevent accidentally overwriting existing indices,
you can check if an index exists with the indexExists
method.
If the source index doesn’t exist, the move
operation is ignored.
Renaming an index doesn’t change the associated analytics:
- Analytics belonging to the original index keep their name.
- A new set of analytics is started with the new name.
See Indices and analytics for more information.
Moving indices with replicas
You can’t move a source index with replicas, as it would break the relationship between the index and its replicas.
You can move an index to a destination index with replicas.
During the process, the data from the destination index is replaced with the data from the source index.
Then, the data is copied to the replicas.